Data structures follow needs. Programmers must learn to assess application needs first, then find a data structure with matching capabilities. To do this requires competence in principles 1, 2, and 3. As I have taught data structures through the years, I have found that design issues have played an ever greater role in my courses. Along with data structures introduction, in real life, problem solving is done with help of data structures and algorithms. An algorithm is a step by step process to solve a problem. In programming, algorithms are implemented in form of methods or functions or routines.
- Data Structures & Algorithms
- Algorithm
- Data Structures
- Linked Lists
- Stack & Queue
- Searching Techniques
- Sorting Techniques
Basic Data Structure Tutorial
- Graph Data Structure
- Tree Data Structure
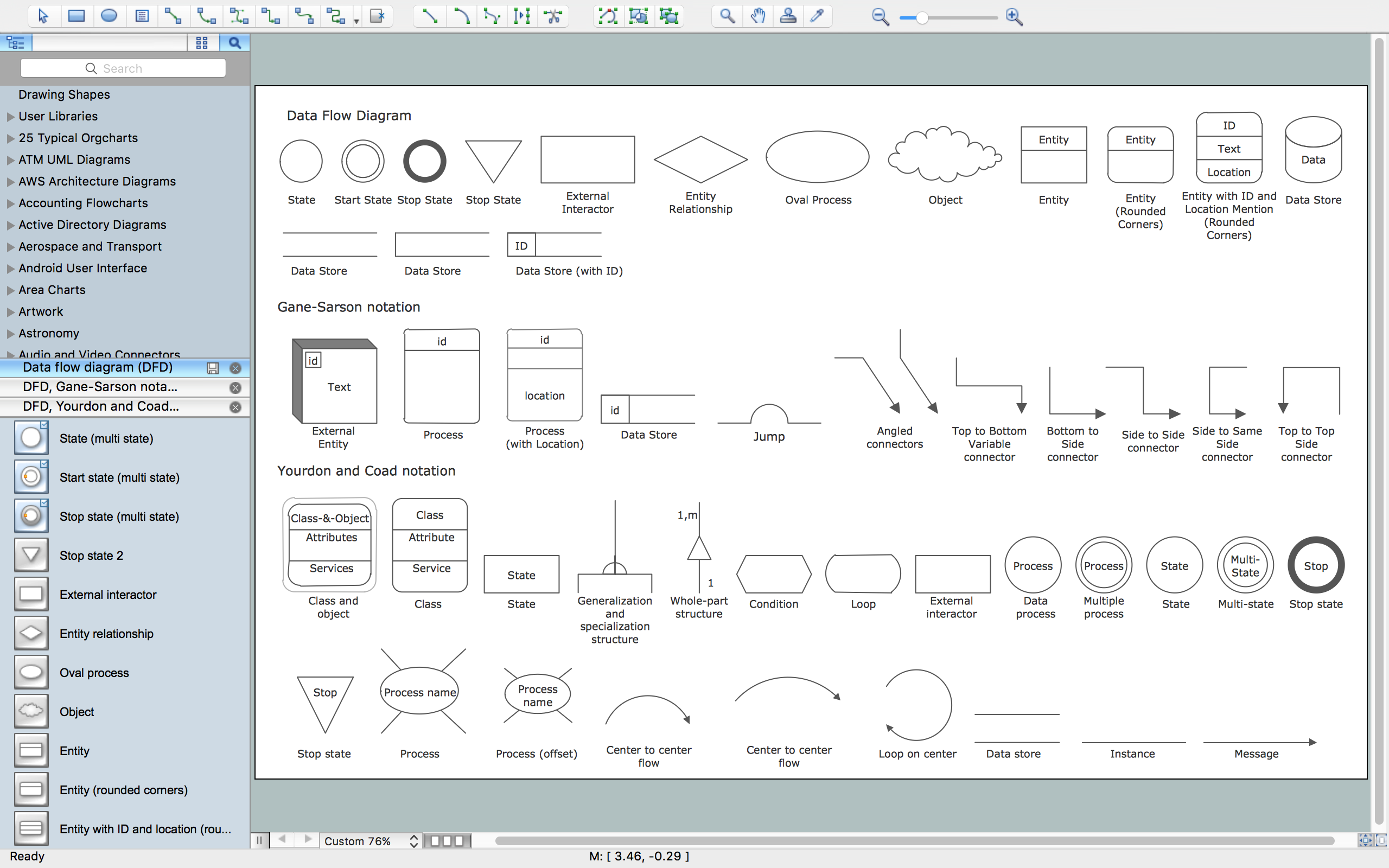
- Recursion
- DSA Useful Resources
- Selected Reading
This chapter explains the basic terms related to data structure.
Data Definition
Data Definition defines a particular data with the following characteristics.

Atomic − Definition should define a single concept.
Traceable − Definition should be able to be mapped to some data element.
Accurate − Definition should be unambiguous.
Clear and Concise − Definition should be understandable.
Data Object
Data Object represents an object having a data.
Data Type
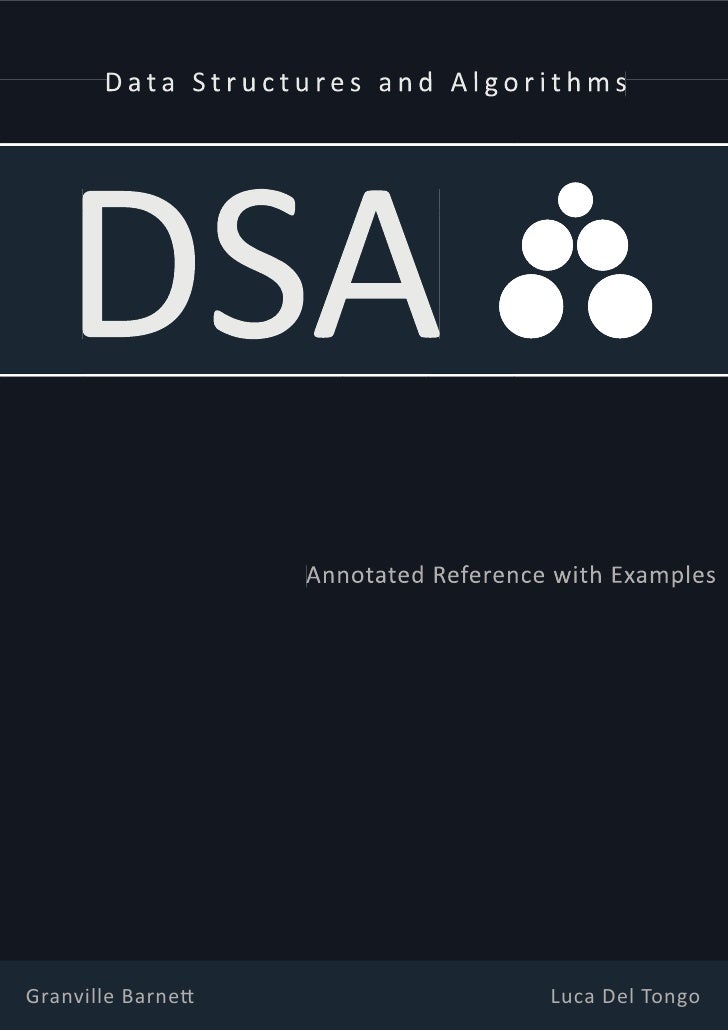
Data type is a way to classify various types of data such as integer, string, etc. which determines the values that can be used with the corresponding type of data, the type of operations that can be performed on the corresponding type of data. There are two data types −
- Built-in Data Type
- Derived Data Type
Built-in Data Type
Those data types for which a language has built-in support are known as Built-in Data types. For example, most of the languages provide the following built-in data types.
- Integers
- Boolean (true, false)
- Floating (Decimal numbers)
- Character and Strings
Derived Data Type
Those data types which are implementation independent as they can be implemented in one or the other way are known as derived data types. These data types are normally built by the combination of primary or built-in data types and associated operations on them. For example −
- List
- Array
- Stack
- Queue
Basic Operations
Data Structures Pdf Free Download
The data in the data structures are processed by certain operations. The particular data structure chosen largely depends on the frequency of the operation that needs to be performed on the data structure.
Use Of Basic Data Structures Pdf Pdf
- Traversing
- Searching
- Insertion
- Deletion
- Sorting
- Merging
- [Instructor] Python provides a number of collection types…useful for creating structured data.…The list type is a basic sequence.…It's created using a pair of square brackets…around a list of values separated by commas.…The list is mutable,…which means that you may add, delete, and change values.…A tuple is like a list, but it's immutable.…You cannot change it once it's been created.…A tuple is created using parentheses.…
A dictionary is a sequence of key-value pairs.…In other languages, this may be called an associative array…or a hashed array.…A dictionary is created using curly brackets.…A set is an unordered list of unique values.…It's useful for finding…and operating upon unique values within a sequence.…A set is indicated with curly brackets.…Any of these collection types…may contain any object or type.…
As we will see in the rest of the chapter,…they may be used to represent simple…or complex structured data.…
Released
1/18/2018 Due to its power and simplicity, Python has become the scripting language of choice for many large organizations, including Google, Yahoo, and IBM. A thorough understanding of Python 3, the latest version, will help you write more efficient and effective scripts. In this course, Bill Weinman demonstrates how to use Python 3 to create well-designed scripts and maintain existing projects. This course covers the basics of the language syntax and usage, as well as advanced features such as objects, generators, and exceptions. Learn how types and values are related to objects; how to use control statements, loops, and functions; and how to work with generators and decorators. Bill also introduces the Python module system and shows examples of Python scripting at work in a real-world application. Topics include:- Python anatomy
- Types and values
- Conditionals and operators
- Building loops
- Defining functions
- Python data structures: lists, tuples, sets, and more
- Creating classes
- Handling exceptions
- Working with strings
- File input/output (I/O)
- Creating modules
- Integrating a database with Python db-api